One of the problems with Visual Basic 6 (VB 6) is its lack of operators. An operator is often ‘syntactic sugar’ – that is, there is another way of expressing the operation using a function call. For example, the C &&
operator (AndAlso
in VB .NET) can be simply replaced by two If
statements. On the other hand, some operations are fundamental in that they are executed by machine instructions – division and multiplication, say. If these aren’t in the language, there’s not much you can do about it.
But there are other operators which, while not in VB 6, can be reasonably easily simulated. Bit shifting is one such. Bit shifts are the movement of the bits in a word or a byte by a given number of places left or right. They aren’t commonly used in database applications, but they are used in cryptography, image processing and communications. The trick to implementing a bit shift function in VB 6 is to realise that it can be implemented by repeatedly using a multiply or divide by 2. Incidentally, in the processor hardware, it’s the other way round – a multiply or divide is performed by repeated bit shifts.
The key idea is that a shift right by one bit is performed by an integer divide by 2, while a shift left is performed by an integer multiply by 2. But having done that, the question remains of what to do with the bit that you have shifted. There are actually two options: either throw it away or ‘rotate’ it into the top or bottom of the word. Here, I’ll ‘rotate’ the bit.
In practice, you have to watch that you don’t cause an ‘overflow’ – a VB 6 exception resulting from the transition from a positive to a negative quantity when you shift to the left. In the code below, I’ve handled this by ‘masking’ the top two bits of a 32 bit word before multiplying (the code shifts a single bit to the left):
The code example in the associated zip file allows you to input a number (in hexadecimal) and shift it by a number of bits. The example actually uses a 64-bit ‘word’ (just an extension of the above example). If you wish, you can extend it to any number of bits in a fairly obvious manner.
Lastly, is this the most efficient way of doing it? Probably not – if you can divide or multiply by 2, you can do so by any power of 2 and so reduce the number of loop iterations. But the cost of this is increased complexity and hence debugging time.
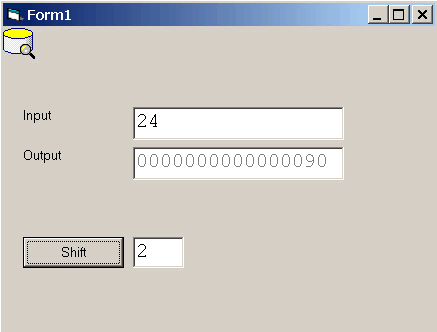
- Just a simple shift left or right. Remember, the input and output are hexadecimal