
- Right-click to download the source code
Part Two of this series
Delphi is a wonderful development system featuring an excellent set of visual design, coding and debugging tools. The principal problem for many programmers is that its Object Pascal language is unfamiliar. Object Pascal is a great language which benefits from a clean and unambiguous syntax. But if you happen to be more familiar with C++, PHP, VB or Java, you may be baffled by numerous simple but important matters such as – well, where do the semicolons go and how do you write a while loop? This series aims to get you over these hurdles to let you start serious Delphi programming as rapidly as possible…
Delphi or Object Pascal? Originally, Delphi was the name of the programming environment and Object Pascal was the name of its language. When Borland added in a mix of other languages, the IDE was renamed The Borland Developer Studio and Delphi became synonymous with the Object Pascal language. Now that the Developer Tools Group are in the process of becoming independent of Borland, the software products have been named Turbo and the version supporting the Object Pascal language is called Turbo Delphi. Many Delphi programmers (myself included) often refer to ‘the Delphi language’. |
Correct Procedures
First, let’s take a look at the structure of a typical Delphi project and some basic Object Pascal syntax. Load up the sample project, multiply.dpr. This implements a very simple calculator which multiplies two values and displays the result. This program doesn’t do much of any interest (we’ve already written more complicated, and useful, calculators in our Introduction To Delphi) – however, the way in which Delphi does it is of interest.
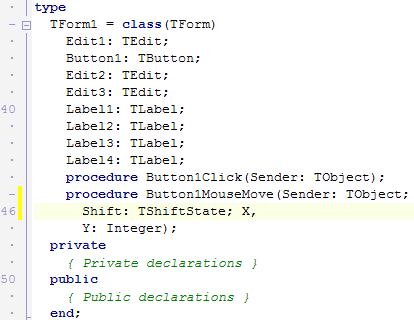
- Delphi writes all this code automatically. But what’s it all mean? Read on…
First, make sure that the form is displayed on screen. You can quickly toggle between the form and code by pressing the F12 function key. Click the ‘Calculate’ button once to select it (if you accidentally double-clicked, you’ll be taken into the code editor, so press F12 to get back to the form view). With the button selected, click the Events tab in the Object Inspector.
This Events page lists all the events – such as keyboard and mouse actions – to which the currently selected control can respond. To create a new event-handler – that is, a code routine which executes when a specific event occurs – you just double-click one of the events in the list.
I’ve already programmed two events for this button. The OnClick event, which is the default event for a button and the OnMouseMove event. Double-click Button1MouseMove, to the right of the OnMouseMove event in the Object Inspector (you may need to scroll down the list to find this) and you will be taken to the relevant code in the editor. This simply displays the mouse coordinates inside the button (these are given by the X and Y arguments which Delphi automatically provides for this procedure) and displays them in the form’s caption whenever the mouse moves over the button itself. I would be the first to admit that this is not a particularly useful thing to do. Let’s see if I can do better with my OnClick event-handler. Press F12 to go back to the form designer. Now double-click the ‘Calculate’ button on the form itself. Since OnClick is the default event for a button, we can locate the code by double-clicking the button as an alternative to selecting the event in the Object Inspector.
The code of the event-handling routine begins with this:
procedure TForm1.Button1Click(Sender: TObject);
In Pascal a procedure is a subroutine which returns no value. A subroutine which returns a value must be declared using the keyword function. I’ll have more to say about procedures and functions in part two of this series.
TForm1 is the type of the form itself and Button1Click() is the name of the event-handling procedure which Delphi automatically creates when you double-click a control on a form or select an event in the Object Inspector. In Object Oriented terms, TForm1 is the ‘class’ of the form and Button1Click() is one of its ‘methods’. The Sender argument is also provided automatically by Delphi and this indicates the control where the original event occurred. In this present procedure, I make use of Sender to display the class type of the control (TButton) in a label on the form:
Label4.Caption := Sender.ClassName;
In real world applications, the Sender parameter could be used for more sensible things – such as determining the origin of a drag-and-drop operation (useful if you want to copy text from one control to another, say).
Let’s take a look at some of the other things that Delphi has ‘written automatically’. Scroll up to the top of the file. First you’ll see that Delphi has named it:
unit mult1;
Every Delphi file lives inside a named unit and your program may use many other units. Then comes the interface keyword. This defines a section of code which is ‘visible’ to any other which uses the current unit. For the time being, you don’t need to be concerned about this (it is explained more fully in the help system) – just be content to let Delphi take care of it for you.
Note: I’ve inserted comments throughout my code. Traditional Pascal comments are placed either between curly braces: { and } or between (* and *). Comments delimited by one type of comment delimiter can be nested inside comments delimited by the other type. Delphi also lets you comment out single lines by putting // characters before anything you wish to comment out. |
The uses section lists those units which are used (‘imported’) by the current program. While you can edit the uses list to add specific units, including those which you have written yourself, for the time being just let Delphi take care of this list for you.
Next comes the type section. Here Delphi has written the type definition, TForm1, of the form itself. Every time you drop a control from the Tool Palette onto the form, Delphi inserts the control’s name and its type into the form definition, like this:
Edit1: TEdit;
If, subsequently, you change the name of a control in the visual designer by setting its Name property in the Object inspector, Delphi will rewrite the underlying code to use the new name. Don’t rewrite the code inside the first part of the definition of TForm1. It is much safer to let Delphi have complete control over this and avoid messing about with it yourself!
Beneath the list of controls, you’ll see that Delphi has also entered two procedure definitions:
procedure Button1Click(Sender: TObject);
procedure Button1MouseMove(Sender: TObject; Shift: TShiftState; X, Y: Integer);
These definitions are required for any ‘methods’ belonging to the form. Where event-handling methods (like these) are concerned, you should let Delphi take care of the writing and rewriting of these entries. If you rename an event in the Events tab of the Object Inspector, Delphi will automatically rewrite the code to match.
The private and public sections of the form definition are available for you to enter your own methods and data. We don’t need to use those for the time being, however (but refer to Delphi Help if you want more information). The class definition terminates with the keyword end;.
Then comes the variable declaration section. This begins with the keyword var. Delphi has inserted a variable, Form1 for the form itself (in OOP terms this an ‘instance’ of the form defined by the TForm1 class definition).
Finally we hit the implementation keyword. Any code routines you write go in this section.
Basic Syntax
When Delphi sets up an event-handling method for you, it creates an empty procedure like this:
procedure TForm1.Button1Click(Sender: TObject);
begin
end;
All you have to do is to edit this by adding the code that you want to be executed. If you want to add some variables, these should be inserted in a section starting with the keyword var just under the procedure header and before the begin. The syntax of variable declaration is described in our Introduction To Delphi. Notice that multiple variables of the same type may optionally be declared one after the other, separated by commas. In other words, this…
var
s1: string;
s2: string;
s3 : string;
…is equivalent to this:
var
s1, s2, s3 : string;
Here is a short summary of some important points of Delphi syntax:
:= |
the assignment operator. |
= |
test for equality. |
<> |
test for ‘not equal’. |
begin |
start a block of code. |
end |
end a block of code. |
; |
terminate a statement or a block of code. |
var |
start declaration of variables. |
if X then |
test condition where X is a true/false expression. |
else |
optional clause after ‘if’ |
’a string’ |
sample string literal enclosed between ’ and ’. |
* |
multiplication operator |
+ |
addition operator. Also string concatenation operator. |
{} |
comment delimiters. |
(**) |
alternative comment delimiters. |
// |
single line comment. |
French Polishing
Let’s look at another example of Delphi coding - a simple French verb conjugator. Before starting on this, you need to know a little about Delphi’s ListBox component. Load up the Prog1.dpr project and find the TForm1.Button1Click procedure in the code editor. This comprises a sequence of statements similar to this:
ListBox1.Items.Add('J''aime' );
As you might expect, this adds the string in parentheses to the items in the ListBox1 object. You don’t need to concern yourself with the exact syntax of this statement just yet. I’ll be looking at similar examples of ‘dot-notation’ later in this series.
It is worth taking a close look at the string itself though. Strings in Delphi are delimited by single-quote characters ‘ and ‘. Notice, however, that the string itself contains two single-quote characters after the initial ‘J’. This is Pascal’s way of placing a single-quote character into a string. As you’ll see, when you run this program, the string that appears in the list box displays only one of these characters:
J’aime
While this little verb conjugator has the makings of a useful program – if you are learning French, anyway – it is currently limited to conjugating just one verb, ‘Aimer’. I really want it to conjugate any verb I ask it to. In Prog2.dpr I’ve made a bit more progress towards this goal. This program lets the user enter any regular ‘-ER’ verb into an edit box and it conjugates this when the button is clicked.
Once again, I’ve done all the coding of this program in the TForm1.Button1Click procedure. As before, this procedure uses an if test when it needs to determine an appropriate action. The test, if verb = ’’, is used to trigger an error message if the Edit box has been left blank. The test, if LowerCase( verbEnd ) <> ’er’, triggers a message if the last two letters of the verb are not ‘er’.
Here LowerCase is the name of a Delphi function. Unlike a procedure, a function returns a value. The LowerCase function returns a string with the same letters as the string passed to it as an argument but with each character now in lowercase. So, for example, LowerCase( ‘HeLLo’ ) would return ‘hello’. |
Each of these if tests also include an else clause which will execute when the test fails (that is, when the Edit box is not blank or when the final letters of the verb are ‘er’). Look closely and you’ll see that the statement immediately prior to the else clause lacks the normal terminating semicolon. In Pascal a semicolon is not permitted before an else clause.
Even though my second attempt at a French verb conjugator is an improvement over my first, it too has deficiencies. For one thing, it completely ignores other French verb forms – the ‘-IR’, ‘-RE’ and irregular verbs. And, for another thing, it fails to contract Je to J’ before a verb that begins with a vowel. I’ll fix those problems in the next article of this series when I’ll be delving a little deeper into the Object Pascal language.